Json방식
Json방식은 매우 중요하다.
json방식? 어떤 데이터를 지정할 때 중괄호로 시작 { }
Json =Java Script Object Notation의 약자 즉 자바스크립트의 객체 표기 방법
만약 d={age:50} // 나이를 50으로 지정한다는 느낌.
d={age:50, name:’이순신‘} // 이 객체가 가지고 있는 나이는 50이고 이름은 이순신이다.
이런식으로 Json방식으로 여러 개 지정도 가능하다.
d=[50,‘이순신’] → 이거는 arrray로 정의한 것
d={age:50, name:’이순신‘} → 이거는 JSon 방식으로 정의한 것
array방식은 50이 몸무게인지 나이인지 전혀 알 수가 없음. 그래서 Json방식을 많이 사용함.
<person>
<age>50</age>
<name>이순신</name>
</person>
이거나
d=[50,‘이순신’]
이거나
d={age:50, name:’이순신‘}
이거나 셋 다 똑같은 의미이지만 셋중에서 마지막 Json방식이 가장 보기쉽고 이해하기도 쉬워서 많이 사용함.
가장 많이 쓰는 방식은 array와 Json을 합쳐서 사용한다.
예를들면
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/js/bootstrap.min.js"></script>
</head>
<body>
<script>
d=[{age:50, name:"이순신"},{age:70, name:"홍길동"}];
alert(d[0].age);
alert(d[0].name);
alert(d[1].age);
alert(d[1].name);
</script>
</body>
</html>
이렇게 쓰면
50→이순신→70→홍길동 순으로 출력된다.
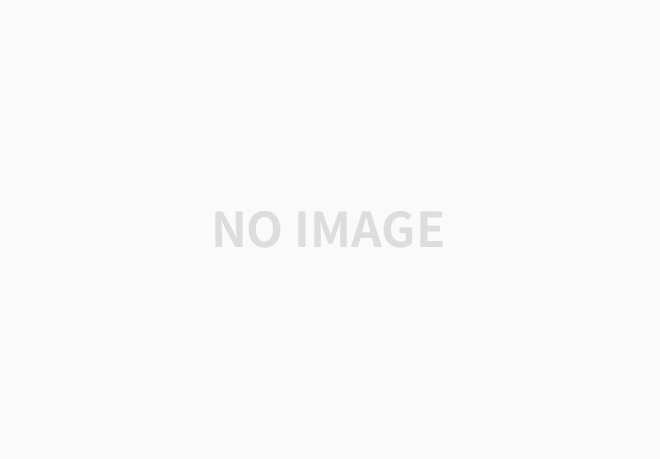
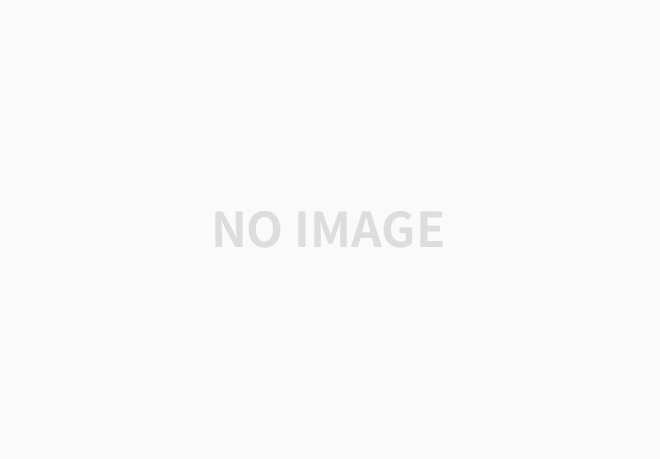
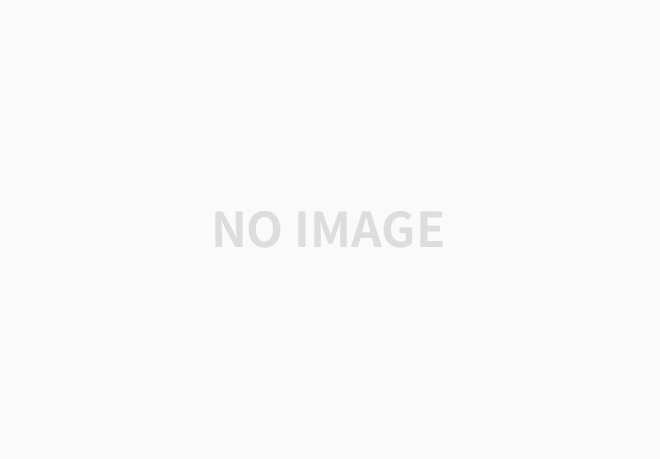
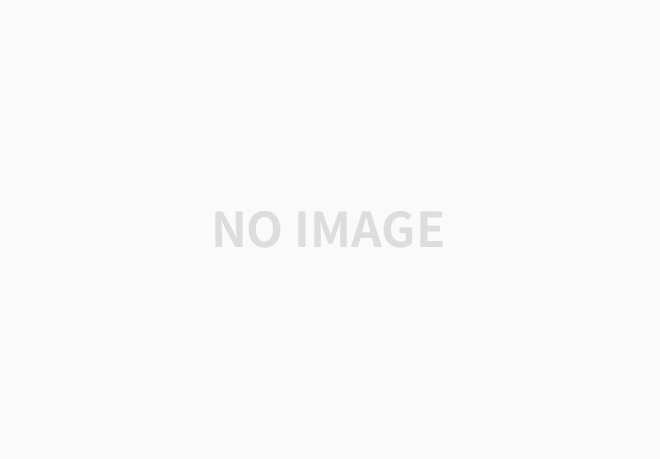
이렇게 출력된다.
웹사이트 출력
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<body>
<script>
a=[0,1,2,3];
var array = [
{ name: 'Hanbit Media', link: 'http://hanb.co.kr' },
{ name: 'Naver', link: 'http://naver.com' },
{ name: 'Daum', link: 'http://daum.net' },
{ name: 'Paran', link: 'http://paran.com' }
];
console.log(array[0].name+" "+ array[0].link);
</script>
</body>
</html>
array 배열중에서 0번째에 해당하는 Hanbit media라는 name을 출력하고 그 이후 " " 이 쌍따옴표 사이의 공백을
집어넣고 그 이후 array 배열중에서 0번째에 해당하는 링크주소를 출력한다.
출력하면 사이트에는 출력되지 않으며, Ctrl+Shift+i로 console창을 띄워 확인할 수 있다.
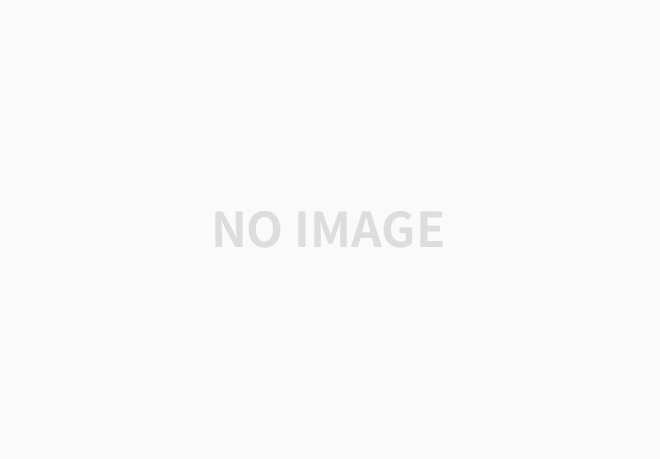
이렇게 나온다.
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<a href=http://www.google.co.kr> google </a> <br>
<a href=http://www.naver.com> naver </a> <br>
<div id="banner">
</div>
<body>
<script>
a=[0,1,2,3];
var array = [
{ name: 'Hanbit Media', link: 'http://hanb.co.kr', image :"movie.jpg" },
{ name: 'Naver', link: 'http://naver.com', image : "food.jpg"},
{ name: 'Daum', link: 'http://daum.net', image : "note1.jpg" },
{ name: 'Paran', link: 'http://paran.com', image : "present.jpg"}
];
console.log(array[0].name+" "+ array[0].link);
html = "";
for(i=0;i<4;i++)
html += "<a href=" + array[i].link +
" ><img src=" + array[i].image +
" height=100>" + array[i].name +
"</a>";
console.log(html);
$("#banner").html(html);
</script>
</body>
</html>
<a href=http://www.google.co.kr> google </a> <br>
<a href=http://www.naver.com> naver </a> <br>
이 부분은 바로 구글과 네이버로 들어가지게 해주는 글자에 하이퍼링크를 입힌 것이고.
사진을 눌렀을 때 해당 사이트로 들어가지게 하는 소스는 스크립트태그 안에있다.
영화 사진 누르면 Hanit media 사이트, 음식 사진 누르면 네이버, 공책사진 누르면 다음,
선물 사진을 누르면 paran 사이트로 들어가지게 설정하였다.
for문을 넣어주어 i값이 0부터 3까지 커질때까지 반복함으로써 array 안의 링크를 전부 출력할수 있게 하였다.
console.log(html);을 넣어 우리가 맞게 했는지 검토할 수 있고
최종 출력은 $("#banner").html(html);덕분에 가능하다.
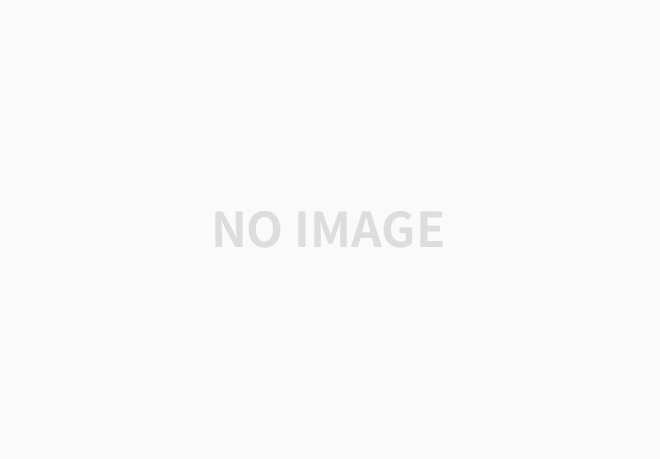
이벤트 연결
<button id="mybtn"> click </button>
$("#mybtn").click(function() {alert('clicked')});
여기가 클릭되면 함수를 실행하세요~
이렇게 click 함수의 파라미터에 함수를 정의하는게 특징.
버튼을 두 개쓸때 다 clicked가 출력되게 하려면
<button id="mybtn"> click </button>
<button id="mybtn"> click2 </button>
$("button").click(function() {alert('clicked')}); 으로 설정해준다.
자바스크립트의 경우 버튼태그에 onclick 이라고 써주고 따로 자바스크립트에서 함수를 정의해주었으나
Jquery로 이렇게 간편하게 단순화 할 수 있음.
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<button id="mybtn"> click </button>
<body>
<script>
$("#mybtn").click(function() {alert('clicked')});
</script>
</body>
</html>
button태그 에서 id를 mybtn으로 설정해주고 JQuery를 이용해 클릭하면 Cliked가 출력되게 하였다.
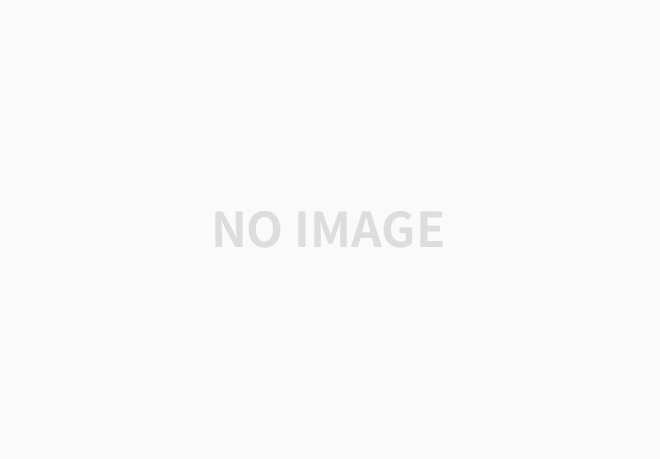
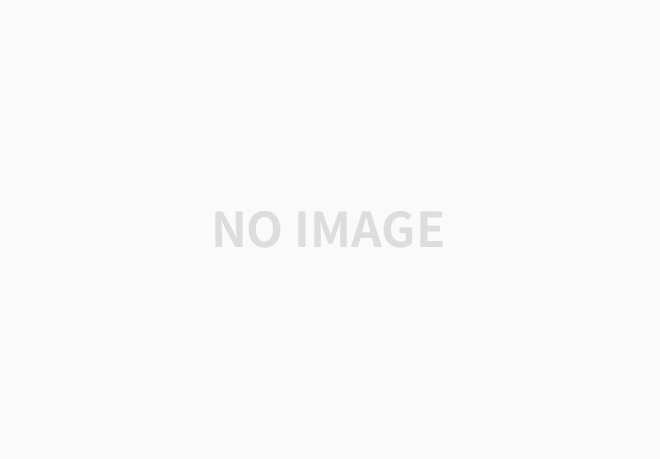
만약 버튼을 2개만들면?
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<button id="mybtn"> click </button>
<button id="mybtn"> click2 </button>
<body>
<script>
$("#mybtn").click(function() {alert('clicked')});
</script>
</body>
</html>
앞에서 우리는 id는 개별로 나타내는 것이라고 배웠다 따라서 click을 누를땐 clicked가 출력되지만
click2를 눌렀을 땐 아무일도 일어나지 않음.
그래서
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<button id="mybtn"> click </button>
<button id="mybtn"> click2 </button>
<body>
<script>
$("button").click(function() {alert('clicked')});
</script>
</body>
</html>
이렇게 버튼이라는 태그를 묶어줘야 click2를 눌러도 clicked가 출력됨. 그런데 보통 이렇게 쓰면 여러개의 버튼이 있을 경우 Clicked가 출력되면 안되는 버튼 까지 clicked가 출력되어 안좋은 코딩임.
그래서
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
<button id="mybtn"> click </button>
<button id="mybtn"> click2 </button>
<button id="mybtn"> click3 </button>
<button id="mybtn"> click4 </button>
<body>
<script>
$("button").click(function() {
alert($(this).text());
});
</script>
</body>
</html>
이렇게 쓰면 내가 클릭을 누른 버튼의 이름에 따라 다르게 출력이 된다.
여기서 핵심은 this인데 this는 이 이벤트를 발생시킨 주체 1을 클릭하면 1, 2를클릭하면 2가 주체가됨
그래서 무엇을 클릭하느냐에 따라 다르게 출력되게 만들어주는 것임.
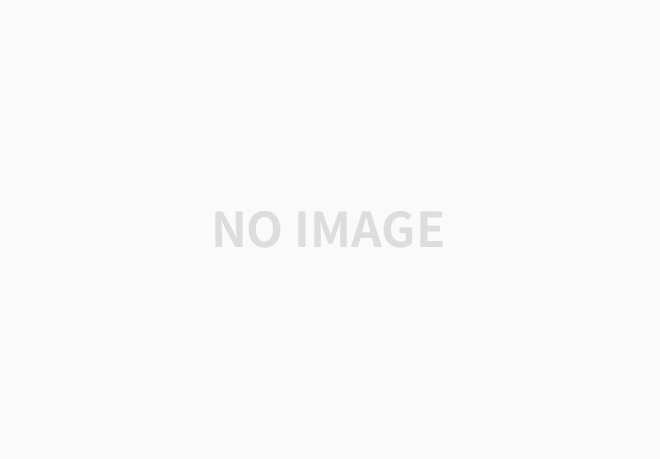
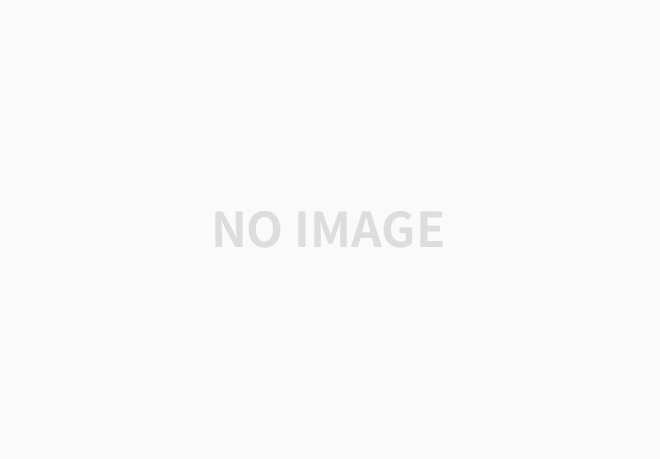
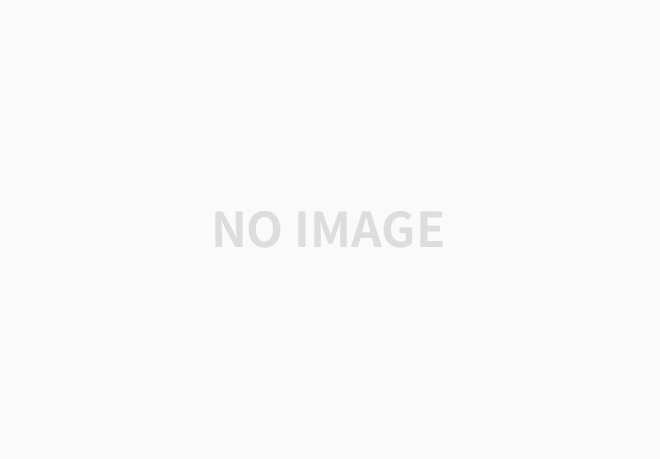
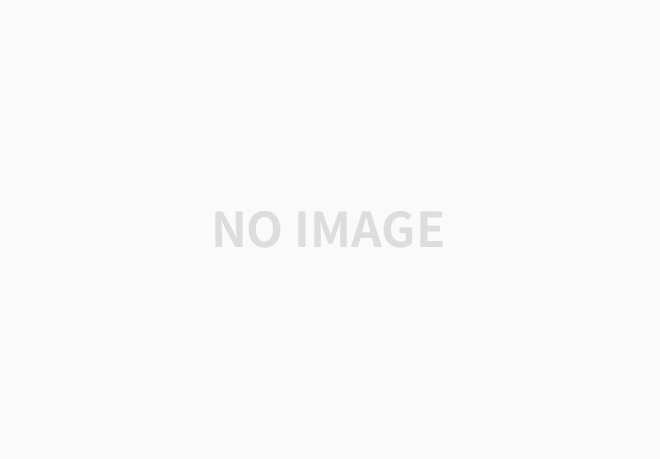
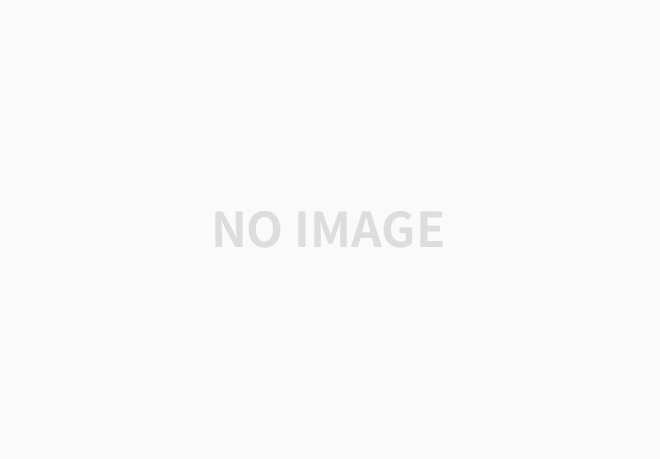
광고 여러개 띄우기
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
광고<br>
<div id="ad">
</div>
<body>
<script>
a=[0,1,2,3];
var array = [
{ name: 'Hanbit Media', link: 'http://hanb.co.kr', image :"movie.jpg" },
{ name: 'Naver', link: 'http://naver.com', image : "food.jpg"},
{ name: 'Daum', link: 'http://daum.net', image : "note1.jpg" },
{ name: 'Paran', link: 'http://paran.com', image : "present.jpg"}
];
idx=Math.floor(Math.random()*array.length); // 난수값 생성 0~3의 난수 생성
ht= "<a href=" + array[idx].link +
" ><img src=" + array[idx].image +
" height=300>" + array[idx].name +
"</a>";
$("#ad").html(ht);
setInterval(change,5000); //5초마다 한번씩 change라는 함수 실행
function change()
{
idx=Math.floor(Math.random()*array.length); // 난수값 생성 0~3의 난수 생성
ht= "<a href=" + array[idx].link +
" ><img src=" + array[idx].image +
" height=300>" + array[idx].name +
"</a>";
$("#ad").html(ht);
} // 5초마다 한번씩 광고 바뀜
</script>
</body>
</html>
굉장히 복잡하게 이루어져 있어 자세학게 설명은 어렵다.
주석을 참고하기 바란다.
대신 이것을 간략화 할 수 있다.
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=0">
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
</head>
광고<br>
<div id="ad">
</div>
<body>
<script>
a=[0,1,2,3];
var array = [
{ name: 'Hanbit Media', link: 'http://hanb.co.kr', image :"movie.jpg" },
{ name: 'Naver', link: 'http://naver.com', image : "food.jpg"},
{ name: 'Daum', link: 'http://daum.net', image : "note1.jpg" },
{ name: 'Paran', link: 'http://paran.com', image : "present.jpg"}
];
setInterval(function() {
idx = Math.floor(Math.random() * array.length);
ht = "<a href=" + array[idx].link +
" ><img src=" + array[idx].image +
" width=300>" + array[idx].name +
"</a>";
$("#ad").html(ht);
}, 5000); // 5초마다 한번씩 광고 바뀜
</script>
</body>
</html>
이렇게 간략화 할 수 있다.
저 두 소스 모두 실행시키면, 처음엔 아무 화면도 나타나지 않다가 5초뒤 갑자기 광고가 생기고
5초뒤 또다른 광고가 생기고 5초마다 계속 랜덤하게 광고가 바뀌게 된다.
'기술노트 > 웹프론트엔드' 카테고리의 다른 글
웹 프론트 엔드 강의(8)(0) | 2020.12.30 |
---|---|
웹 프론트 엔드 강의(7)(0) | 2020.12.29 |
웹 프론트 엔드 강의(5)(0) | 2020.12.28 |
웹 프론트엔드 강의(4)(0) | 2020.12.25 |
웹 프론트엔드 강의(3)(0) | 2020.12.25 |